String/Object in Array Testing in Java – using Arrays.asLi
- 时间:2020-10-07 14:38:56
- 分类:网络文摘
- 阅读:99 次
This tutorial presents three ways of testing if a given element (integer, doubles, objects, strings) is in a given Array using Java programming language.
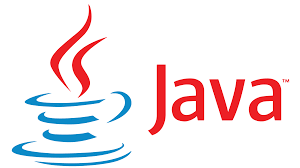
Java
Java’s Naive Implementation of Element (String) In Array Tests using Generic
In Java, if we want to test a given element in array, we can implement a naive version (let’s take String for example):
1 2 3 4 5 6 | private static boolean stringInArray1(String str, String[] arr) { for (var x: arr) { if (x.equals(str)) return true; } return false; } |
private static boolean stringInArray1(String str, String[] arr) { for (var x: arr) { if (x.equals(str)) return true; } return false; }
If we want to go with generic type, we can of course:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | package com.helloacm; public class Main<T> { private boolean inArray(T val, T[] arr) { for (T x: arr) { if (x.equals(val)) return true; } return false; } public static void main(String[] args) { var arr = new String[] {"a", "ab", "abc", "abcde"}; var test = new Main<String>(); System.out.println(test.inArray(null, arr)); System.out.println(test.inArray("adfasd", arr)); System.out.println(test.inArray("a", arr)); } } |
package com.helloacm; public class Main<T> { private boolean inArray(T val, T[] arr) { for (T x: arr) { if (x.equals(val)) return true; } return false; } public static void main(String[] args) { var arr = new String[] {"a", "ab", "abc", "abcde"}; var test = new Main<String>(); System.out.println(test.inArray(null, arr)); System.out.println(test.inArray("adfasd", arr)); System.out.println(test.inArray("a", arr)); } }
In-Array Test using Arrays.AsList().contains
We can convert the array type to List using Arrays.asList then in this case, we can simply use its contain method:
1 2 3 | private boolean stringInArray1(T val, T[] arr) { return Arrays.asList(arr).contains(val); } |
private boolean stringInArray1(T val, T[] arr) { return Arrays.asList(arr).contains(val); }
Parallel version of Element-In-Array Tests using Arrays.Stream
Even better, we can convert the array to stream, then make a parallel version of the in-array test. It is cool, and it’s potentially faster!
1 2 3 4 5 | private boolean stringInArray1(T val, T[] arr) { return Arrays.stream(arr). parallel(). anyMatch(s -> s.equals(val)); } |
private boolean stringInArray1(T val, T[] arr) { return Arrays.stream(arr). parallel(). anyMatch(s -> s.equals(val)); }
–EOF (The Ultimate Computing & Technology Blog) —
推荐阅读:称螺丝的数学题 一乘二分之一加二乘三分之一加…九十九乘一百分之一加一百乘一百零一分之一等于多少?求答题过程和答案。 为什么所有剩下的钱加起来是51元? 十二个乒乓球特征相同,其中只有一个重量异常 某银行有两种储蓄计划——单利和复利的问题 大院里养了三种动物。每只小山羊戴3个铃铛,每只狗戴1个铃铛 某本书正文所标注的页码共用了2989个阿拉伯数字,问这本书的正文一共有多少页? 有两棵古槐树,500年前有个学者说 高中生儿童节作文 勿以恶小而为之勿以善小而不为
- 评论列表
-
- 添加评论