How to Convert Float Number (Fraction) to Hexadecimal in Python?
- 时间:2020-09-28 16:28:51
- 分类:网络文摘
- 阅读:77 次
We known that in Javascript, we can use the toString(16) to convert an integer to its hexadecimal representation. That works even for float numbrs, for example,
1 2 3 4 5 6 | (0.5).toString(16) "0.8" (1.5).toString(16) "1.8" (0.3).toString(16) "0.4ccccccccccccc" |
(0.5).toString(16) "0.8" (1.5).toString(16) "1.8" (0.3).toString(16) "0.4ccccccccccccc"
In python, you can do this using the following function FloatToHex that will print at most (by default) 16 decimal places in hexadecimal form.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | import sys def FloatToHex(x, k = 16): if x < 0: sign = "-" x = -x else: sign = "" s = [sign + str(int(x)) + '.'] x -= int(x) for i in range(k): y = int(x * 16) s.append(hex(y)[2:]) x = x * 16 - y return ''.join(s).rstrip('0') if __name__ == "__main__": print(FloatToHex(float(sys.argv[1]))) |
import sys def FloatToHex(x, k = 16): if x < 0: sign = "-" x = -x else: sign = "" s = [sign + str(int(x)) + '.'] x -= int(x) for i in range(k): y = int(x * 16) s.append(hex(y)[2:]) x = x * 16 - y return ''.join(s).rstrip('0') if __name__ == "__main__": print(FloatToHex(float(sys.argv[1])))
Examples:
# python3 FloatToHex.py 0.5 0.8 # python3 FloatToHex.py 0.6 0.99999999999998 # python3 FloatToHex.py 0.3 0.4ccccccccccccc # python3 FloatToHex.py 2.3 2.4cccccccccccc # python3 FloatToHex.py -2.3 -2.4cccccccccccc
The complexity is O(1) if we consider the string manipulation is also O(1) and the hex function in Python runs at O(1) constant.
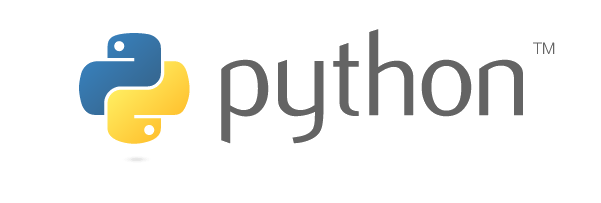
python
–EOF (The Ultimate Computing & Technology Blog) —
推荐阅读:4 Frequently Discussed SEO Myths Exposed 3 Reasons Why Graphic Designers Need to Self-Promote through Ins How to Split a String in C++? The Best Instagram Feed WordPress Plugins to Use Trie Class Data Structure in Python How to Monitor the CPU Temperature of Raspberry PI using Python Listen to what an SEO expert has to say about its benefits Depth First Search Algorithm to Compute the Smallest String Star Algorithm to Check if All Points are On the Same Line Prefix Sum Algorithm to Count Number of Nice Subarrays
- 评论列表
-
- 添加评论