How to Convert Set to Vector in C++?
- 时间:2020-09-27 14:36:16
- 分类:网络文摘
- 阅读:87 次
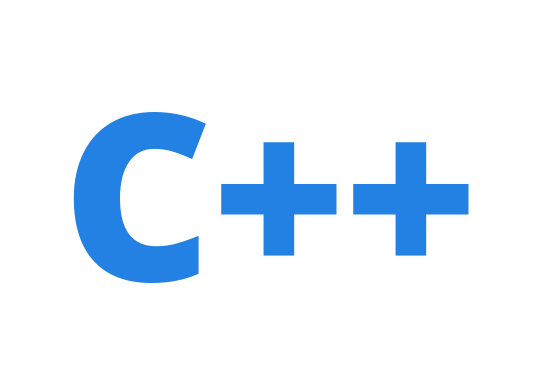
cplusplus
Let’s say our task is to convert a set or unordered_set in C++ to std::vector, what would you do?
Given the following set with integers (or other types)
1 | unordered_set<int> data; |
unordered_set<int> data;
We want to convert it to:
1 | vector<int> target; |
vector<int> target;
Using a Loop to copy a C++ set to std::vector
Intuitively, we can push to the vector one by one, in a loop, by reading the elements in the set.
1 2 3 4 | vector<int> target; for (const auto &it: data) { target.push_back(it); } |
vector<int> target; for (const auto &it: data) { target.push_back(it); }
Alternatively, we can pre-allocate the size of the vector, and use something like this (slightly a bit faster):
1 2 3 4 | vector<int> target(data.size()); for (int i = 0; i < data.size(); ++ i) { target[i] = data[i]; } |
vector<int> target(data.size()); for (int i = 0; i < data.size(); ++ i) { target[i] = data[i]; }
Using vector constructor to convert a C++ set to std::vector
In the constructor of the vector, we can pass the iterator of begin and end for the input set, like below:
1 | vector<int> target(data.begin(), data.end()); |
vector<int> target(data.begin(), data.end());
Using vector.assign to convert a C++ set to std::vector
We can use the std::vector.assign() method that takes the begin and end iterator of the source set/unordered_set – which will allow us to convert/copy a C++ set to std::vector, like below.
1 2 | vector<int> target; target.assign(data.begin(), data.end()); |
vector<int> target; target.assign(data.begin(), data.end());
Using std::copy to copy a C++ set to std::vector
Using std::copy, we can specify the begin and end iterator of the source data to copy from, and the begin iterator of the target data source, like this:
1 2 | vector<int> target(data.size()); std::copy(data.begin(), data.end(), target.begin()); |
vector<int> target(data.size()); std::copy(data.begin(), data.end(), target.begin());
We have to make sure the target data has enough space/storage to copy the data to, thus, the target vector has to be pre-allocated.
Alternatively, we can use the back_inserter that will insert at the end of the target vector, thus no need to allocate the target vector.
1 2 | vector<int> target; std::copy(data.begin(), data.end(), std::back_inserter(res)); |
vector<int> target; std::copy(data.begin(), data.end(), std::back_inserter(res));
–EOF (The Ultimate Computing & Technology Blog) —
推荐阅读:牛奶和酸奶,到底哪个更有营养呢? 健康与饮食:养胃护胃之八大饮食禁忌 关于饮用牛奶饮品的六个健康误区 不同颜色的玉米其营养价值也各不相同 富含膳食纤维蔬菜之红薯的保健作用 红薯怎么吃润肠通便及红薯食用禁忌 吃海带的十大好处和海带的饮食禁忌 经常食用这三种饭有助于预防肠癌 保健食品需谨慎 所谓效果是心理作用 关于预防癌症的十条饮食和生活建议
- 评论列表
-
- 添加评论