Javascript’s toFixed Implementation without Rounding
- 时间:2020-09-24 11:54:15
- 分类:网络文摘
- 阅读:67 次
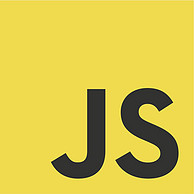
NodeJs / Javascript
In Javascript, you can use Number.prototype.toFixed(n) that will return a string version that has n decimal places. For example,
1 2 | (3).toFixed(3) // "3.000" (3.15).toFixed(3) // "3.150" |
(3).toFixed(3) // "3.000" (3.15).toFixed(3) // "3.150"
The toFixed will round up the last digit (from 6 not 5):
1 2 | (3.1595).toFixed(3) // "3.159" (3.1596).toFixed(3) // "3.160" |
(3.1595).toFixed(3) // "3.159" (3.1596).toFixed(3) // "3.160"
If we don’t want to round-up the decimal values, we can to this thanks to Regular Expression Matching:
1 2 3 4 5 6 7 8 9 10 | Number.prototype.toFixedNoRounding = function(n) { const reg = new RegExp("^-?\\d+(?:\\.\\d{0," + n + "})?", "g") const a = this.toString().match(reg)[0]; const dot = a.indexOf("."); if (dot === -1) { // integer, insert decimal dot and pad up zeros return a + "." + "0".repeat(n); } const b = n - (a.length - dot) + 1; return b > 0 ? (a + "0".repeat(b)) : a; } |
Number.prototype.toFixedNoRounding = function(n) { const reg = new RegExp("^-?\\d+(?:\\.\\d{0," + n + "})?", "g") const a = this.toString().match(reg)[0]; const dot = a.indexOf("."); if (dot === -1) { // integer, insert decimal dot and pad up zeros return a + "." + "0".repeat(n); } const b = n - (a.length - dot) + 1; return b > 0 ? (a + "0".repeat(b)) : a; }
The idea is to convert the number into string (via toString()) and use the RegExp to truncate the extra zeros (without rounding). Next, we find out the position of decimal dot (if any), and pad up the zeros for exactly n decimal places. We compute the number of zeros we need and if it is positive, we use the String.prototype.repeat() to insert additional zeros at the end. We could also use the String.prototype.padEnd() which may seem a bit less intuitive.
1 2 3 4 5 6 7 | console.log((3.1999).toFixedNoRounding(3)); // 3.199 console.log((3.19923413412349).toFixedNoRounding(3)); // 3.199 console.log((3.01).toFixedNoRounding(3)); // 3.010 console.log((3.01).toFixedNoRounding(4)); // 3.0100 console.log((3.01).toFixedNoRounding(5)); // 3.01000 console.log((3).toFixedNoRounding(3)); // 3.000 console.log((-3).toFixedNoRounding(3)); // -3.000 |
console.log((3.1999).toFixedNoRounding(3)); // 3.199 console.log((3.19923413412349).toFixedNoRounding(3)); // 3.199 console.log((3.01).toFixedNoRounding(3)); // 3.010 console.log((3.01).toFixedNoRounding(4)); // 3.0100 console.log((3.01).toFixedNoRounding(5)); // 3.01000 console.log((3).toFixedNoRounding(3)); // 3.000 console.log((-3).toFixedNoRounding(3)); // -3.000
–EOF (The Ultimate Computing & Technology Blog) —
推荐阅读:小学生日记端午节 天天感恩节|小学作文 中秋节英语 难忘的一次中秋节 森林艺术节 各地的清明节习俗 艺术节的演出 清明节缅怀先烈 校园艺术节 秋天是一个美丽的梦
- 评论列表
-
- 添加评论